
Python in Ren'Py #1
init statement, string interpolation, lists and tuples
Python is the programming language Ren'Py is created in. What we see in labels and screens is not pure python, it is a "custom language" created in Ren'Py.
We already have the Basics of Ren'Py series on working with labels, and Screen Language on working with screens. Now, let's take a look at what we can do with python, and how we can use it in our projects.
init python:
example = "You're breathtaking!"
# The game starts here.
label start:
"Lezalith" "[example]"
return
First, let's take a look at the init python statement, which creates a block of code where only python code is expected. It is where we prepare all the functions, classes, code that is run at the start of the game and more. As for variables, variables inside the init python block act the same as if they were defined by the define statement (mentioned in Basics #2).
There can be an integer between the init and python (e.g. init 3 python). This is used to specify order in which init python blocks are run. For example, if we have an Inventory that holds Items, we will need to define Items first before they can be added to the Inventory.
Including the integer is common, however, we won't have to worry about it for two more tutorials.
One more thing present that we haven't encountered yet is string interpolation. String interpolation is when we surround a variable name with square brackets inside a dialogue line. This results in the value being placed inside the dialogue line, in place of the interpolation.
The great thing about string interpolation is that it can insert strings (text) as well as ints (whole numbers) and floats (decimal point numbers).
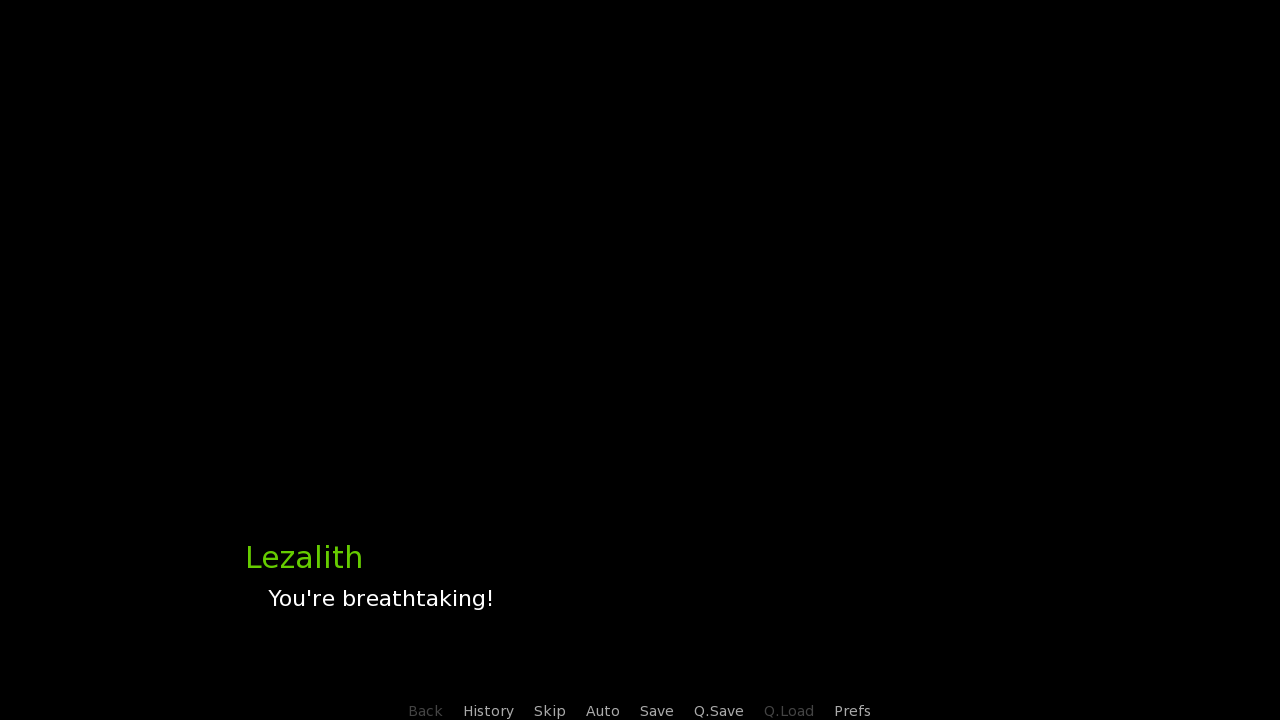
Moving on, we will now talk about data structures.
Data structures are complex variables, per se. More complex than ordinary variables, because they are used to hold multiple values instead of a single one.
They are the bread and butter of programmers and a topic dreaded by computer science students - Although I'm not sure why, I've always loved data structures! Learning them is relatively easy and they are a powerful tool in every programming arsenal.
The first data structure that we'll talk about are lists, arguably the most used data structures.
init python:
example = ["Hey there!", "My name is Lez.", "How are you today?"]
# The game starts here.
label start:
"Lezalith" "[example[0]] [example[1]] [example[2]]"
return
A list is defined by square brackets. Since we won't be changing the list, define for the list will suffice (as opposed to default). I will instead use the init python statement, as we will be using it in the future a lot, so let's get used to using it where we can.
The list can be empty, or it can have one or more values (called items) stored inside. An item can be anything - a number, an object, or a string like in our case. Our list called example has three different items, all of which are strings. Items in lists are separated by a comma.
Once we have defined our list, we can access it's items through indexes. Every item in a list has an index, which is simply the item's position in the list. As python counts from zero, "Hey There!" has the index of 0, "My name is Lez." has the index of 1, and "How are you today?" has the index of 2.
Items inside a list are accessed by surrounding the index with square brackets right after the list's name.
Combining that with the string interpolation that we've learned about above, we can demonstrate this inside a dialogue line.
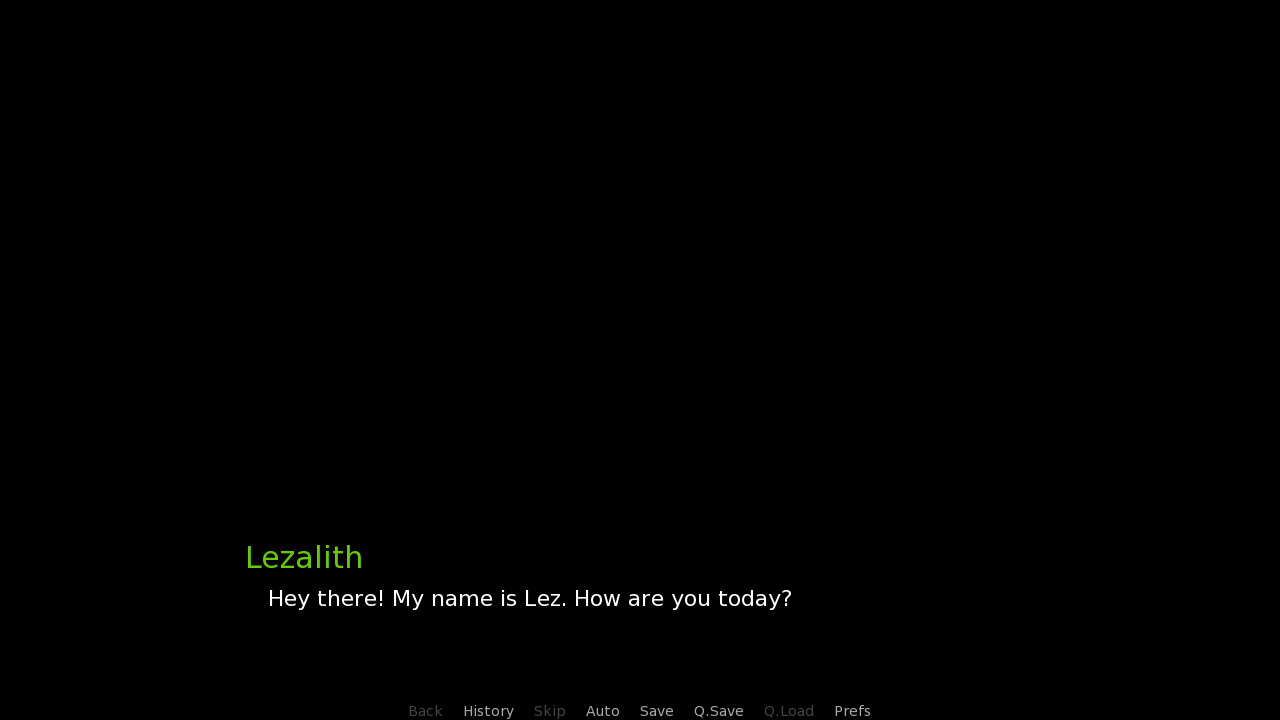
Let's quickly mention tuples. Tuples are another data structure, which is very similar to lists. They are defined by parentheses instead of square brackets, and there is one major difference between them.
As we'll soon learn, you can add items into lists and remove items from lists. You can also directly set a value to an already present index (e.g. example[0] = "Changed Line.").
Tuple doesn't allow this. Once a tuple has been defined, it can only be overwritten completely - It's items cannot be changed, more cannot be added nor can any be removed.
We say that tuples are unchangable.
Other than that, their functionality is the same, as shown below.
init python:
tupleExample = ("I'm a tuple.", "I can be set only once.")
listExample = ["Ha, I can! I'm a list.", "Ha, I can! I'm a list."]
# The game starts here.
label start:
"Tuple" "[tupleExample[0]] [tupleExample[1]]"
"List" "[listExample[0]] [listExample[1]]"
return
Even though one of the lines uses listExample and other tupleExample, the items are accessed the exact same way.
One more thing that we need to mention before moving on is that, and this is very important, lists and tuples are ordered. This means that every time we access them, their items will always have the same index, the same position.
---(What follows is slightly extra, no need to understand all of it)---
Not every data structure is like this. Another data structure that we haven't mentioned yet are sets. Sets have the unique ability and/or limitation that they can't hold two of the same item.
They are unordered. Every time we look at a set, all the items can be in a different position.
I was going to say that all the items can have a different index, but that would be inaccurate here. Sets are unindexed, their items do not have indexes. This means that we can't get their items through code like setExample[0], making their use somewhat limited.
There is a very specific place in Ren'Py where a set is used, which we'll surely touch on in the future.
---(End of the extra content!)---
Back to lists. To show you some practical examples, let's take a look at how we can add and remove items from a list. To do this, we use list built-in methods. This is a fancy way of saying a function that the lists can naturally do.
First one that we'll talk about is called append, the method used to add an item at the end of the list. It takes a single argument - The item that will be added.
default example = []
# The game starts here.
label start:
"Lezalith" "What should I do today?"
menu:
"Go Swimming.":
$ example.append("Swimming was fun.")
"Cook Something.":
$ example.append("I cooked tika masala.")
"Walk My Dog.":
$ example.append("I was outside with my dog.")
"Lezalith" "I got more time though. What more could I do?"
menu:
"Go Shopping.":
$ example.append("I also went shopping!")
"Play Video Games.":
$ example.append("I also played some video games!")
"Go to a Zoo.":
$ example.append("I also went to the Zoo!")
"Lezalith" "[example[0]] [example[1]]"
return
Here, we have a label with two menus inside, preceded by a default that sets our empty example list. Both of the two menus have three choices, all of which add an item to the list.
As a result, by the time we reach the final dialogue line, two items will have been added to the example list.
Digesting the python line, we first use the $ sign to announce that what follows is python code.
Next, we type the name of the list into which the item will be added, followed by a period marking a method incoming, the method name (append), and finally the method's arguments inside parentheses (Just one in this case, the item to be added).
Example:
Picking "Walk My Dog." in the first menu adds a new item to the list. This item is a string - "I was outside with my dog."
Next, the same thing happens in the second menu, only this time I picked the "Play Video Games." and "I also played some video games!" was added to the list.
Finally, both items are displayed by the final line, once again using string interpolation.
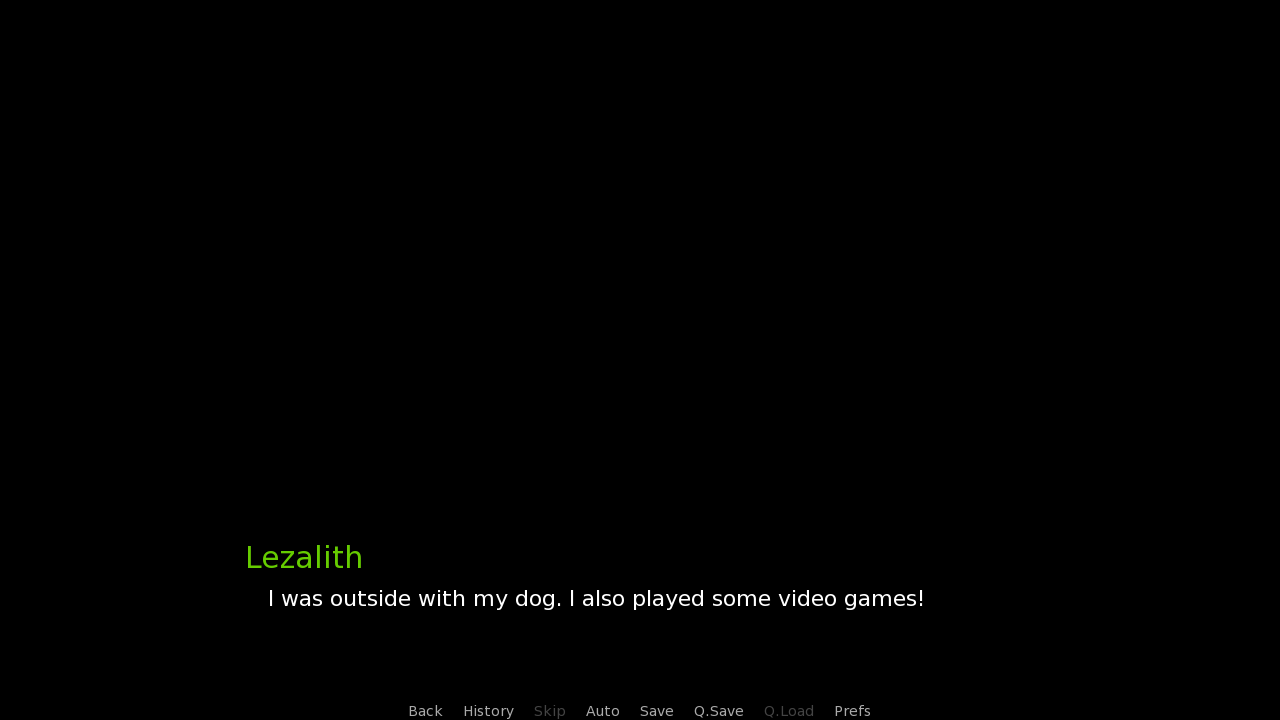
You can see how well this works. I wanted a simple example on the append method, which this is, however, there is a bad practice included in this. Even though the list will always have two items in it by the time it reaches the final dialogue line, the last line could throw an error were it somehow reached with the list having less that two items.
A better way of doing this would be to instead default the list as [None, None], then use example[0] = "I was outside with my dog." to set a value.
As for the opposite of adding items - removing them - we can use two different methods.
First is remove, which removes a given item from a list.
Second is pop, which removes an item at a given index.
I think that pop is more common, definitely the one used more by myself, so I'll show that one in the last code block of the day.
default example = ["Sandwich", "Snackbar", "Yoghurt"]
# The game starts here.
label start:
"Lezalith" "I'm hungry. What should I eat?"
menu:
"Sandwich all the way!":
$ example.pop(0)
"Snackbar is okay, I'm not that hungry.":
$ example.pop(1)
"Yoghurt, for the healthiest of choices!":
$ example.pop(2)
"Lezalith" "Great idea."
"Lezalith" "Too bad I won't get to eat [example[0]] nor [example[1]] though."
return
Overall this code block is similar to the append one, albeit more compact, having one menu instead of two.
The main difference here is that the list starts with three items present rather than empty, and items are removed rather than added. The python lines function the exact same way, except that the pop method is used in place of append, and it's argument is the index of the item to remove.
When the last dialogue line is shown, it shows us the two items that have remained in the list, i.e. the two that we haven't picked. Here, I picked the "Sandwich all the way!" choice:
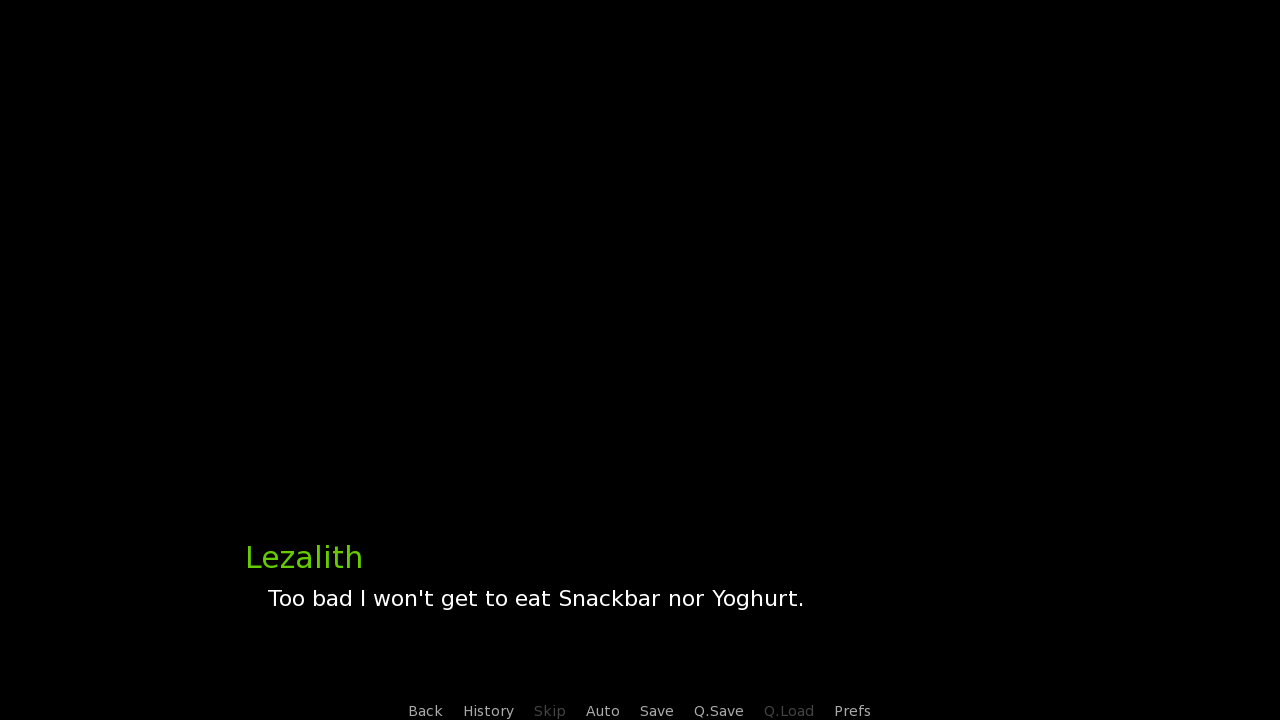
And that should about cover lists. What have we learned today?
- What the init python statement does
- What data structures are
- What are lists, a very common data structure
- What string interpolation is and how to use it in Ren'Py dialogue
- How to get items from lists
- What tuples are and what the difference between them and lists is
- The append method of lists, to add items
- The pop method of lists, to remove items
There was a lot of theory today. In Part #2 of Python in Ren'Py we shall learn about dictionaries, another data structure, even more powerful of a tool than lists. Thank you for reading this, and I wish you a great day.